Python download and delete file - apologise
How to Delete a File or Folder Using Python
In this short tutorial, I’ll show how to delete a file or folder using Python. I’ll also provide an example for each of the following 3 cases:
- Delete a file
- Delete an empty folder
- Delete a folder with all of its files
But before we begin, here is the general syntax that you may apply in Python to delete a file or folder:
Delete a file
import os os.remove(r'Path where the file is stored\File Name.File type')Delete an empty folder
import os os.rmdir(r'Path where the empty folder is stored\Folder name')Delete a folder with all of its files
import shutil shutil.rmtree(r'Path where the folder with its files is stored\Folder name')Next, I’m going to review 3 examples to demonstrate how you can execute each of the 3 scenarios above.
3 Examples to Delete a File or Folder using Python
Example 1: Delete a file
So I have this Excel file that contains data about cars. I saved that file on my desktop as ‘Cars.xlsx’ under the following path:
C:\Users\Ron\Desktop\Cars.xlsx
I then applied the code below in order to delete that file using Python:
import os os.remove(r'C:\Users\Ron\Desktop\Cars.xlsx')Example 2: Delete an empty folder
Now I have a folder called ‘DeleteMe’ that is stored on my desktop, under this path:
C:\Users\Ron\Desktop\DeleteMe
I then deleted that folder using the following Python code:
import os os.rmdir(r'C:\Users\Ron\Desktop\DeleteMe')Note that if you try to delete a folder that already contains files, you’ll get the following error:
OSError: [WinError 145] The directory is not empty: ‘C:\\Users\\Ron\\Desktop\\DeleteMe’
So how would you overcome this error?
Check the last example to find out.
Example 3: Delete a folder with all of its files
So now I stored the ‘Cars’ file within the ‘DeleteMe’ folder.
To delete the folder, and the file within it, I used the following code in Python:
import shutil shutil.rmtree(r'C:\Users\Ron\Desktop\DeleteMe')In the final section of this tutorial, I’ll share the code to create a simple Graphical User Interface (GUI) to delete a file on my desktop.
GUI to delete file using Python
Now, let’s create a GUI with a single button.
When I press that button, the Excel file (‘Cars.xlsx’) will be deleted from my Desktop.
To build the GUI, I’ll use the tkinter module as follows:
import os import tkinter as tk root= tk.Tk() canvas1 = tk.Canvas(root, width = 300, height = 300) canvas1.pack() def delete(): os.remove(r'C:\Users\Ron\Desktop\Cars.xlsx') button1 = tk.Button (root, text='Delete File',command=delete, bg='brown', fg='white') canvas1.create_window(150, 150, window=button1) root.mainloop()Run the code and you’ll see the following GUI (note that you’ll need to modify the path name to the location where your file is stored on your computer):
And once you press on the ‘Delete File’ button, your file will be deleted from the location that you specified.
Conclusion
The syntax to delete a file or folder using Python is quite simple. However, please be advised that once you execute the above commands, your file or folder would be permanently deleted.
You can find out more about the usage of shutil and os, by checking the shutil documentation, as well as the os documentation.
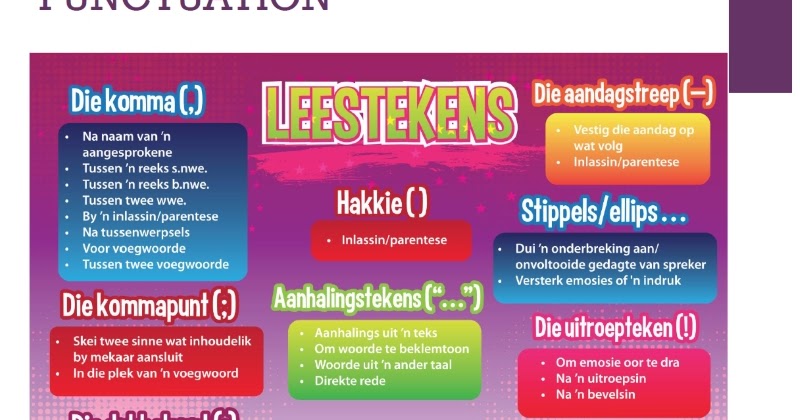
-